Table of Contents
Introduction
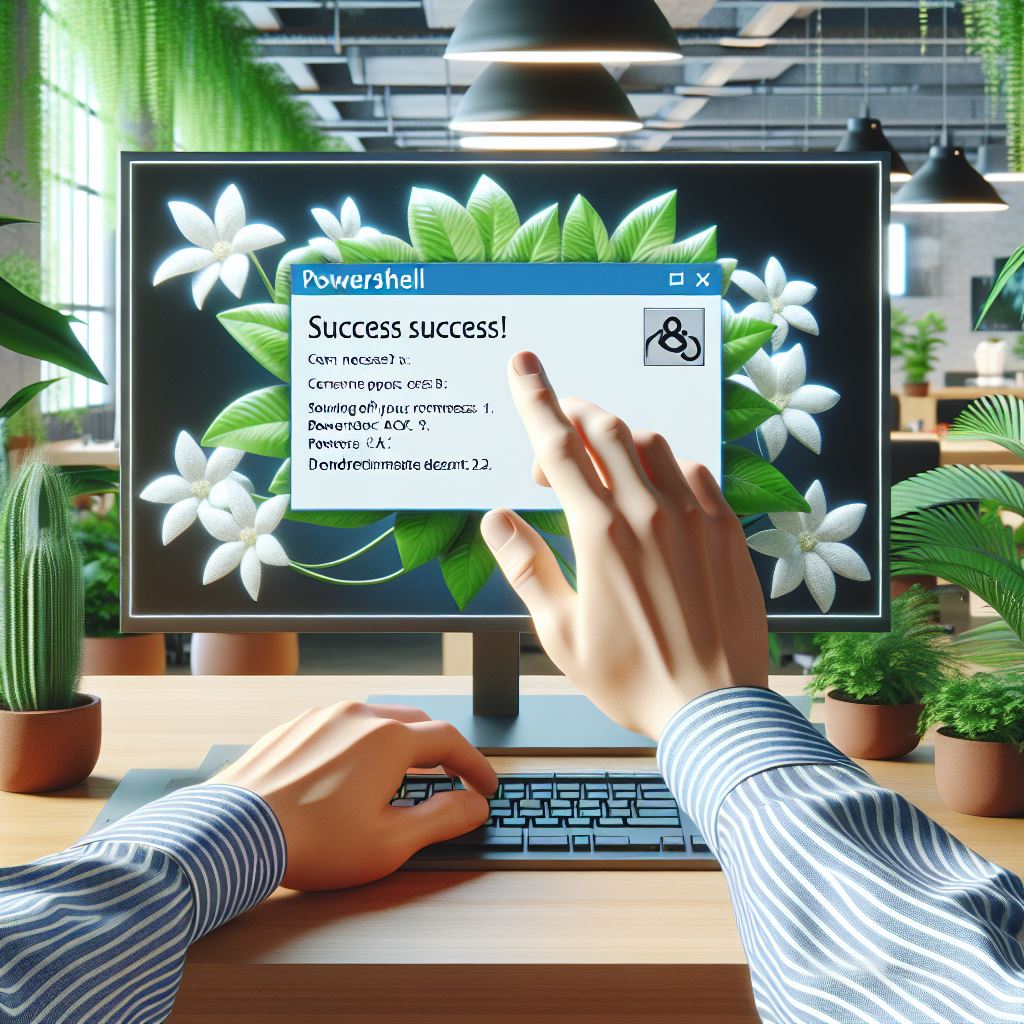
Welcome to our guide on using PowerShell to interact with the User Information List in SharePoint Online. In this guide, we’ll provide practical examples of PowerShell commands that allow you to access, modify, and manage user data within the User Information List.
Read more: The Hidden User List in SharePoint Online
PowerShell Commands
Connect to SharePoint Online
Install the SharePoint Online Management Shell: If you haven’t already, install the SharePoint Online Management Shell to use the Remove-SPOUser
cmdlet.
Install-Module -Name Microsoft.Online.SharePoint.PowerShell
Install the PnP PowerShell Module: If you haven’t already, install the PnP PowerShell module to use the Remove-PnPUser
cmdlet.
Install-Module -Name SharePointPnPPowerShellOnline
Connect to SharePoint Online: Use the Connect-SPOService
or Connect-PnPOnline
cmdlets to connect to your SharePoint Online environment.
# Connect to SharePoint Online
$adminSiteUrl = "https://yourtenant-admin.sharepoint.com"
Connect-SPOService -Url $adminSiteUrl
Or
# Connect to SharePoint Online
$siteUrl = "https://yourtenant.sharepoint.com/sites/yoursite"
Connect-PnPOnline -Url $siteUrl -UseWebLogin
Execute the Commands: Run the appropriate command based on your needs.
Retrieve User Information
Use the Get-SPOUser
cmdlet to retrieve user information from a specific site collection:
# Retrieve user information from the site collection
$siteUrl = "https://yourtenant.sharepoint.com/sites/yoursite"
$userLoginName = "[email protected]"
# Get user information
$user = Get-SPOUser -Site $siteUrl -LoginName $userLoginName
# Display user information
Write-Host "Display Name: $($user.DisplayName)"
Write-Host "Email: $($user.Email)"
Write-Host "Login Name: $($user.LoginName)"
Write-Host "Department: $($user.Department)"
Write-Host "Job Title: $($user.JobTitle)"
Retrieve All Site Users and Export to CSV
Use the Connect-PnPOnline
cmdlet to establish a connection to your SharePoint Online site:
# Connect to SharePoint Online
$siteUrl = "https://yourtenant.sharepoint.com/sites/yoursite"
Connect-PnPOnline -Url $siteUrl -UseWebLogin
Use the Get-PnPUser
cmdlet to retrieve all users from the site and export the information to a CSV file:
# Retrieve all users from the site
$users = Get-PnPUser
# Select the properties you want to export
$userData = $users | Select-Object DisplayName, Email, LoginName, Department, JobTitle
# Export the user data to a CSV file
$userData | Export-Csv -Path "C:\Path\To\Export\Users.csv" -NoTypeInformation
Retrieve All SharePoint Groups and Export to CSV
Use the Get-PnPGroup
cmdlet to retrieve all groups from the site and export the information to a CSV file:
# Retrieve all groups from the site
$groups = Get-PnPGroup
# Select the properties you want to export
$groupData = $groups | Select-Object Title, Id, Description
# Export the group data to a CSV file
$groupData | Export-Csv -Path "C:\Path\To\Export\SharePointGroups.csv" -NoTypeInformation
Retrieve Group Members and Export to CSV
Use the Get-PnPGroupMembers
cmdlet to retrieve members of a specific group:
# Parameters
$siteUrl = "https://yoursharepointsiteurl"
$groupName = "Your SharePoint Group Name"
$outputFile = "C:\path\to\output.csv"
# Connect to SharePoint Online
Connect-PnPOnline -Url $siteUrl -UseWebLogin
# Get the group
$group = Get-PnPGroup -Identity $groupName
# Get group members
$members = Get-PnPGroupMember -Group $group
# Export to CSV
$members | Select-Object DisplayName, Email | Export-Csv -Path $outputFile -NoTypeInformation
Write-Host "Group members exported to $outputFile"
Retrieve All Sites, Site Groups,Group Permissions, Group Members and Export to CSV
# Parameters
$outputFile = "C:\path\to\output.csv"
# Connect to SharePoint Online
Connect-PnPOnline -Url "https://yoursharepointadminsiteurl" -UseWebLogin
# Get all site collections
$siteCollections = Get-PnPTenantSite
# Initialize an array to store the results
$results = @()
foreach ($site in $siteCollections) {
# Connect to each site collection
Connect-PnPOnline -Url $site.Url -UseWebLogin
# Get all groups in the site collection
$groups = Get-PnPGroup
foreach ($group in $groups) {
# Get group members
$members = Get-PnPGroupMember -Group $group
# Get group permissions
$permissions = Get-PnPGroupPermissions -Identity $group
foreach ($member in $members) {
$results += [PSCustomObject]@{
SiteUrl = $site.Url
GroupName = $group.Title
Permission = $permissions
MemberName = $member.DisplayName
MemberEmail = $member.Email
}
}
}
}
# Export to CSV
$results | Export-Csv -Path $outputFile -NoTypeInformation
Write-Host "Data exported to $outputFile"
Retrive users in SharePoint Online sites who no longer exist in Azure Active Directory (AAD)
Make sure you have the PnP PowerShell module and AzureAD module installed and configured. You can install them using:
Install-Module SharePointPnPPowerShellOnline
Install-Module AzureAD
This script connects to your SharePoint Online tenant, retrieves all site collections and users, checks if each user exists in Azure AD, and exports the data of users who no longer exist in AAD to a CSV file.
# Parameters
$outputFile = "C:\path\to\output.csv"
# Connect to SharePoint Online
Connect-PnPOnline -Url "https://yoursharepointadminsiteurl" -UseWebLogin
# Get all site collections
$siteCollections = Get-PnPTenantSite
# Initialize an array to store the results
$results = @()
foreach ($site in $siteCollections) {
# Connect to each site collection
Connect-PnPOnline -Url $site.Url -UseWebLogin
# Get all users in the site collection
$users = Get-PnPUser
foreach ($user in $users) {
# Check if the user exists in AAD
$aadUser = Get-AzureADUser -ObjectId $user.Email -ErrorAction SilentlyContinue
if (-not $aadUser) {
$results += [PSCustomObject]@{
SiteUrl = $site.Url
UserName = $user.Title
UserEmail = $user.Email
}
}
}
}
# Export to CSV
$results | Export-Csv -Path $outputFile -NoTypeInformation
Write-Host "Data exported to $outputFile"
Add a User to a SharePoint Group
Use the Add-SPOUser
cmdlet to add a user to a specific SharePoint group within a site collection:
# Define variables
$siteUrl = "https://yourtenant.sharepoint.com/sites/yoursite"
$groupName = "YourGroupName"
$userLoginName = "[email protected]"
# Add the user to the specified group
Add-SPOUser -Site $siteUrl -Group $groupName -LoginName $userLoginName
Create a New Group
Use the New-PnPGroup
cmdlet to create a new group in the site:
# Define variables
$groupName = "NewGroupName"
$groupDescription = "Description of the new group"
# Create the new group
New-PnPGroup -Title $groupName -Description $groupDescription -Owner "[email protected]"
Remove a User from a Site Collection
To remove a user from a specific site collection, you can use the Remove-SPOUser
cmdlet. This cmdlet requires the user’s login name and the site collection URL.
# Connect to SharePoint Online
Connect-SPOService -Url https://yourtenant-admin.sharepoint.com
# Remove the user from the site collection
Remove-SPOUser -Site https://yourtenant.sharepoint.com/sites/yoursite -LoginName [email protected]
Remove a User from All Site Collections
Use the Connect-SPOService
cmdlet to establish a connection to your SharePoint Online environment:
# Connect to SharePoint Online
$adminSiteUrl = "https://yourtenant-admin.sharepoint.com"
Connect-SPOService -Url $adminSiteUrl
Use the Remove-SPOUser
cmdlet to remove the user from each site collection:
# Define the user to be removed
$userLoginName = "[email protected]"
# Loop through each site collection and remove the user
foreach ($site in $siteCollections) {
Write-Host "Removing user from site collection: $($site.Url)"
Remove-SPOUser -Site $site.Url -LoginName $userLoginName
}
Remove a User from a Group
If you need to remove a user from a specific group within a site collection, you can specify the group name along with the user’s login name.
# Connect to SharePoint Online
Connect-SPOService -Url https://yourtenant-admin.sharepoint.com
# Remove the user from the group
Remove-SPOUser -Site https://yourtenant.sharepoint.com/sites/yoursite -Group "Group Name" -LoginName [email protected]
Remove a Group from a Site Collection
Use the Remove-SPOSiteGroup
cmdlet to remove a group from a specific site collection:
# Define variables
$siteUrl = "https://yourtenant.sharepoint.com/sites/yoursite"
$groupName = "GroupToRemove"
# Remove the group from the site collection
Remove-SPOSiteGroup -Site $siteUrl -Identity $groupName
Remove a User from the User Information List
To remove a user from the User Information List, you can use the Remove-PnPUser
cmdlet from the PnP PowerShell module. This cmdlet requires the user’s login name and the site URL.
# Connect to SharePoint Online
Connect-PnPOnline -Url https://yourtenant.sharepoint.com/sites/yoursite -UseWebLogin
# Remove the user from the User Information List
Remove-PnPUser -LoginName [email protected]
Force user synchronization between Azure Active Directory (AAD) and SharePoint Online
Install the Required Modules
Install the necessary modules for Azure AD and SharePoint Online:
Install-Module -Name MSOnline
Install-Module -Name Microsoft.Online.SharePoint.PowerShell
Connect to Azure AD and SharePoint Online
Use the Connect-MsolService
and Connect-SPOService
cmdlets to establish connections to Azure AD and SharePoint Online:
# Connect to Azure AD
Connect-MsolService
# Connect to SharePoint Online
$adminSiteUrl = "https://yourtenant-admin.sharepoint.com"
Connect-SPOService -Url $adminSiteUrl
Force User Synchronization
Use the PeopleManager class from the SharePoint Client Object Model (CSOM) to synchronize user profile properties:
# Load SharePoint CSOM libraries
Add-Type -Path "C:\Program Files\Common Files\microsoft shared\Web Server Extensions\16\ISAPI\Microsoft.SharePoint.Client.dll"
Add-Type -Path "C:\Program Files\Common Files\microsoft shared\Web Server Extensions\16\ISAPI\Microsoft.SharePoint.Client.Runtime.dll"
Add-Type -Path "C:\Program Files\Common Files\microsoft shared\Web Server Extensions\16\ISAPI\Microsoft.SharePoint.Client.UserProfiles.dll"
# Function to synchronize user profile properties
function Sync-UserProfileProperties {
[string]$siteUrl,
[string]$userLoginName
)
# Connect to SharePoint Online
$context = New-Object Microsoft.SharePoint.Client.ClientContext($siteUrl)
$context.Credentials = Get-Credential
# Get PeopleManager instance
$peopleManager = New-Object Microsoft.SharePoint.Client.UserProfiles.PeopleManager($context)
# Get user profile properties from Azure AD
$userProfile = $peopleManager.GetPropertiesFor($userLoginName)
$context.Load($userProfile)
$context.ExecuteQuery()
# Update user profile properties in SharePoint Online
$peopleManager.SetSingleValueProfileProperty($userLoginName, "Department", $userProfile.UserProfileProperties["Department"])
$peopleManager.SetSingleValueProfileProperty($userLoginName, "Title", $userProfile.UserProfileProperties["Title"])
$context.ExecuteQuery()
Write-Host "User profile properties synchronized for $userLoginName"
}
# Example usage
$siteUrl = "https://yourtenant.sharepoint.com/sites/yoursite"
$userLoginName = "[email protected]"
Sync-UserProfileProperties -siteUrl $siteUrl -userLoginName $userLoginName
Disclaimer
This code is provided as is without warranty of any kind, either express or implied, including any implied warranties of fitness for a particular purpose, merchantability, or non-infringement.
Lastest Posts
- Microsoft Teams: Dedicated download option for tracking all registrations in Webinar [MC1131062]
- SharePoint Agent: New Agent Link WebPart [MC1131063]
- Microsoft Purview | New centralized Service health dashboard for Microsoft Purview [MC1131059]
- Microsoft Lens app will retire [MC1131064]
- New admin policy controls for video creation and distribution in Microsoft Clipchamp and Microsoft Stream [MC1131077]